Introduction to Java Server Pages JSP
This tutorial is the first one of several where we will see what are JSP pages, how they work and their main uses, as well as its related technologies.
What is a JSP?
A JSP is a document based in HTMLs tags and in its own tags. Below we will see an example of a page called login.jsp:
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<title>Login result</title>
</head>
<body>
<%
String user = request.getParameter("user");
String pass = request.getParameter("password");
if ("edu4java".equals(user) && "eli4java".equals(pass)) {
out.println("login ok");
} else {
out.println("invalid login");
}
%>
</body>
</html>
Here we can see that all the code is HTML except for what is inside the tags <% %> which is java code. This JSP does the same as the code in the following Servlet.
package com.edu4java.servlets; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class LoginServlet extends HttpServlet { @Override protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String user = request.getParameter("user"); String pass = request.getParameter("password"); if ("edu4java".equals(user) && "eli4java".equals(pass)) { response(response, "login ok"); } else { response(response, "invalid login"); } } private void response(HttpServletResponse resp, String msg) throws IOException { PrintWriter out = resp.getWriter(); out.println("<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\"" + " \"http://www.w3.org/TR/html4/loose.dtd\">"); out.println("<html>"); out.println("<head>"); out.println("<meta http-equiv=\"Content-Type\" content=\"text/html; " + "charset=ISO-8859-1\">"); out.println("<title>Login result</title>"); out.println("</head>"); out.println("<body>"); out.println("<t1>" + msg + "</t1>"); out.println("</body>"); out.println("</html>"); } }
From the point of view of a web page designer, the jsp is a lot more simple than a servlet. Taking into account that normally in the companies, the web designers don´t have great knowledge on java and vice versa, it is obvious the advantages of the JSP. If there is a lot of html on the page and there is a lot of work, it can become a nightmare, even for the most senior java developer. Imagine pages and pages of out.println( ...
<%
String user = request.getParameter("user");
String pass = request.getParameter("password");
if ("edu4java".equals(user) && "eli4java".equals(pass)) {
out.println("login ok");
} else {
out.println("invalid login");
}
%>
If we take a look at the java code of the JSP we can notice that there is no declaration of the variables "request" and "out". These variables are known as Implicit Objects and there are predefined with standard names to be used by the programmer in the java code of the JSPs. Below there is a description of some of these Implicit Objects:
request | object with the information received from the browser. |
response | object which will contain the information that we will send back as a response to the browser. |
out | object Writer which we can use to send Html (or any another type of content) to the browser. |
session | object which keeps the data in between calls of the same user. |
application | object which keeps the data during all the life of the application. |
The objects request y response are the ones which are received as parameters in the methods doPost(HttpServletRequest request, HttpServletResponse response) and doGet(HttpServletRequest request, HttpServletResponse response). The objects request, response and application allows us to keep information and we will see them in detail in a tutorial on "web application scope".
Testing login.jsp
We copy login.jsp into WebContent and we modify login.html so that instead of calling /login, it calls directly to login.jsp
<html>
<body>
<form action="login.jsp" method="post">
<table>
<tr>
<td>User</td>
<td><input name="user" /></td>
</tr>
<tr>
<td>password</td>
<td><input name="password" /></td>
</tr>
</table>
<input type="submit" />
</form>
</body>
</html>
Click on the right button of the mouse in login.html - Run as - Run on server. http://localhost:8080/first-jee/login.html is open. We fill user, password and we click on the Submit Query button.
Another advantage of the JSPs, is that it is not necessary to declare them in the web.xml.
How does a JSP work in the server o Servlet container?
When we deploy a JSP and start a server, the server creates the java code for a servlet based on the JSP content and it compiles it. In my installation we can find the files .java and .class of this servlet in:
C:\eclipseJEE2\workspace\.metadata\.plugins\org.eclipse.wst.server.core\tmp0\work\Catalina\localhost\first-jee\org\apache\jsp
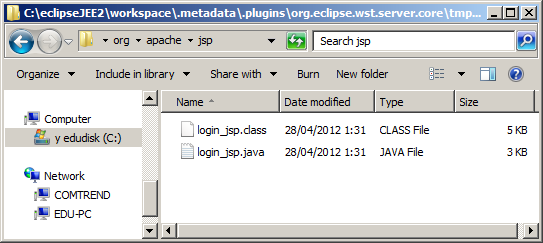
It is not necessary to understand all the generated java code but it is interesting to have a look at it.
package org.apache.jsp; import javax.servlet.*; import javax.servlet.http.*; import javax.servlet.jsp.*; public final class login_jsp extends org.apache.jasper.runtime.HttpJspBase implements org.apache.jasper.runtime.JspSourceDependent { private static final JspFactory _jspxFactory = JspFactory.getDefaultFactory(); private static java.util.List _jspx_dependants; private javax.el.ExpressionFactory _el_expressionfactory; private org.apache.AnnotationProcessor _jsp_annotationprocessor; public Object getDependants() { return _jspx_dependants; } public void _jspInit() { _el_expressionfactory = _jspxFactory.getJspApplicationContext(getServletConfig() .getServletContext()).getExpressionFactory(); _jsp_annotationprocessor = (org.apache.AnnotationProcessor) getServletConfig() .getServletContext().getAttribute(org.apache.AnnotationProcessor.class.getName()); } public void _jspDestroy() { } public void _jspService(HttpServletRequest request, HttpServletResponse response) throws java.io.IOException, ServletException { PageContext pageContext = null; HttpSession session = null; ServletContext application = null; ServletConfig config = null; JspWriter out = null; Object page = this; JspWriter _jspx_out = null; PageContext _jspx_page_context = null; try { response.setContentType("text/html"); pageContext = _jspxFactory.getPageContext(this, request, response, null, true, 8192, true); _jspx_page_context = pageContext; application = pageContext.getServletContext(); config = pageContext.getServletConfig(); session = pageContext.getSession(); out = pageContext.getOut(); _jspx_out = out; out.write("<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\""+ " \"http://www.w3.org/TR/html4/loose.dtd\">\r\n"); out.write("<html>\r\n"); out.write("<head>\r\n"); out.write("<meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\">\r\n"); out.write("<title>Login result</title>\r\n"); out.write("</head>\r\n"); out.write("<body>\r\n"); out.write("\t"); String user = request.getParameter("user"); String pass = request.getParameter("password"); if ("edu4java".equals(user) && "eli4java".equals(pass)) { out.println("login ok"); } else { out.println("invalid login"); } out.write("\r\n"); out.write("</body>\r\n"); out.write("</html>"); } catch (Throwable t) { if (!(t instanceof SkipPageException)){ out = _jspx_out; if (out != null && out.getBufferSize() != 0) try { out.clearBuffer(); } catch (java.io.IOException e) {} if (_jspx_page_context != null) _jspx_page_context.handlePageException(t); } } finally { _jspxFactory.releasePageContext(_jspx_page_context); } } }
Some interesting things:
- The class HttpJspBase extends from HttpServlet.
- _jspService(HttpServletRequest request, HttpServletResponse response) replaces doPost and doGet.
- We can see the declarations of the implicit objects request, response, out, session, etc.
- The html code of our JSP is sent to the browser using out.write().
In the next tutorial we are going to see how to debug our web aplication..
<< Web application Deployment | Web application debugging>> |